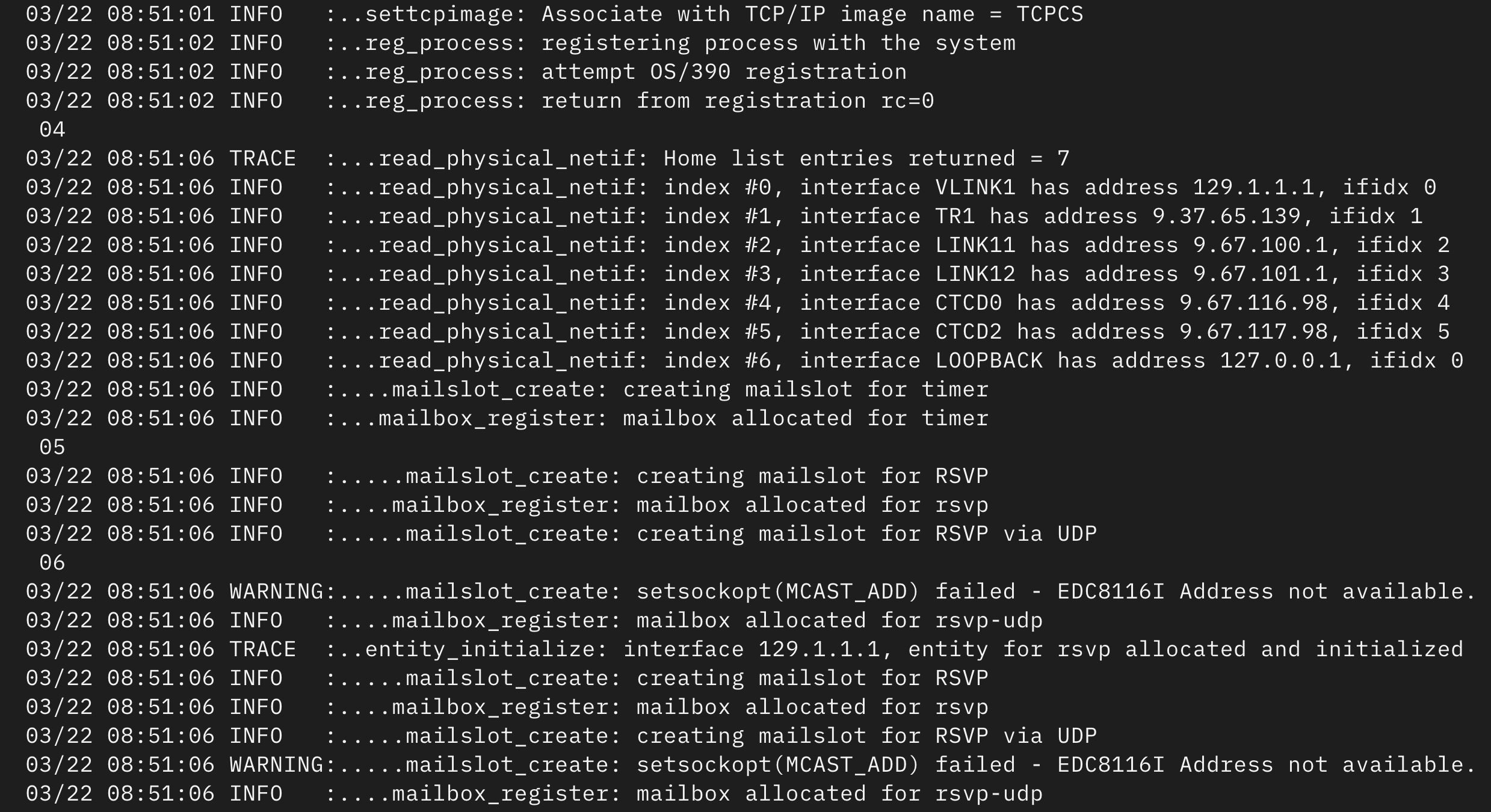
I tend to look at a lot of log output throughout the day as part of my role as platform engineer. This obviously extends to any backend, or ops-related, role. One little niggle that always gets to me is tailing output where the lines only change when something interesting happens.
I have no idea if time is actually passing. Obviously, I can eyeball timestamps if available, but there are times when so many lines zip through the buffer that it’s easy to lose track or even go cross-eyed.
It can be tricky to notice that lines are still being tailed if the output doesn’t drastically change in some meaningful or noticeable way. Something I like to is intercept each line and then output some kind of divider whenever n
lines have been added to the buffer.
It’s as simple as:
#!/usr/bin/env bash
lines=0
while IFS= read -r line; do
echo "${line}"
((lines++))
if ((lines % 5 == 0)); then
echo "----------"
fi
done
Effectively, all this does is:
- Intercept each line of output being piped into the script.
- Keep a running tally of lines we’ve intercepted so far;
$lines++
. - If the current tally is divisible by
n
, or in this case5
, spit out an additional line containing a divider. - Keep doing this forever until the process is terminated.
If you were to save this in a file named as divider.sh
and set it to execute with something like chmod a+x
you could test it by doing something like:
$ while true; do echo "emitting a noop"; sleep 1; done | ./divider.sh
And you’ll see something like the following:
emitting a noop
emitting a noop
emitting a noop
emitting a noop
emitting a noop
----------
emitting a noop
emitting a noop
emitting a noop
emitting a noop
emitting a noop
----------
This is pretty dumb, but now you can see that output is still being placed in your terminal buffer. I’ve set it to every 5
lines, but you can update the script to make that configurable. You could also play a sound when the script places a divider in the buffer using something like tput bel
.
Anyway… Enjoy.